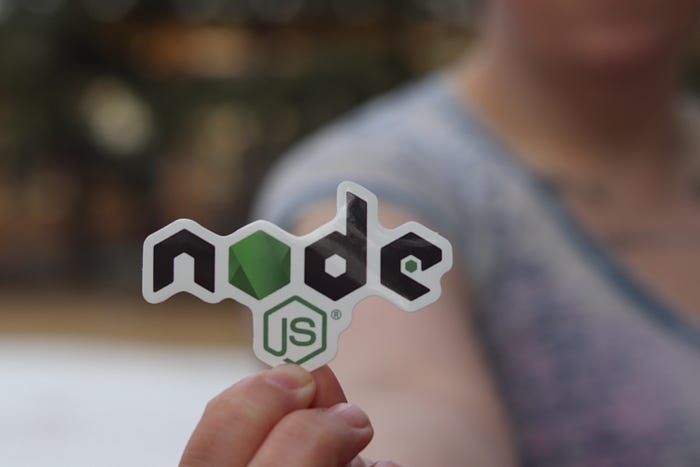
Node.js is a powerful runtime environment for executing JavaScript code on the server side. There are several popular frameworks available for Node.js that help developers build web applications efficiently. Some notable Node.js frameworks:
- Express.js
- Nest.js
- Koa.js
- Hapi.js
here we are looking in to the basics to key difference of most popular frameworks Express.js and Nest.js.
Web server using Express and Nest
Here’s a simple JavaScript program that uses the Express framework to create a basic web server.
// Import the required modules
const express = require('express');
// Create an instance of the Express application
const app = express();
// Define a router for the root URL
app.get('/', (req, res) => {
res.send('Hello World!');
});
// Start the server on port 3000
app.listen(3000, () => {
console.log('Server is running on port 3000');
});
Below is an example of a simple JavaScript server using Nest.js, a popular Node.js framework for building scalable and maintainable application.
Step 1: Install the Nest CLI globally by running the following command in your terminal:
npm install -g @nestjs/cli
Step 2: Create a new Nest.js project by running the following command:
nest new my-nest-app
Step 3: Navigate to the project directory:
cd my-nest-app
Step 4: Open the src/main.ts
file and replace its content with the following code:
import { NestFactory } from '@nestjs/core';
import { AppModule } from './app.module';
async function bootstart() {
const app = await NestFactory.create(AppModule);
await app.listen(3000);
console.log('Server is running on http://localhost:3000');
}
bootstart();
Step 5: Create a new file src/app.controller.ts
with the following code:
import { Controller, Get } from '@nestjs/common';
@Controller()
export class AppController {
@Get()
getHello(): string {
return 'Hello, World!';
}
}
Step 6: Open the src/app.module.ts
file and modify it as follows:
import { Module } from '@nestjs/common';
import { AppController } from './app.controller';
@Module({
controllers: [AppController],
})
export class AppModule {}
Step 7: Finally, start the server by running the following command:
npm run start
Key difference between Express and Nest
Express.js and Nest.js are both popular frameworks for building web applications with Node.js, but they have some key differences in their design philosophy and features. Here’s a comparison between Express.js and Nest.js.
Scalability and Architecture
- Express.js: Express.js provides a lightweight and flexible foundation, making it suitable for small to medium-sized applications. However, as the application grows, developers have more responsibility to structure and organize the codebase.
- Nest.js: Nest.js promotes a modular and scalable architecture from the start. It encourages the use of modules, services, and controllers to achieve a structured and maintainable codebase. Nest.js also provides features like module encapsulation and separation of concerns to handle larger and complex applications effectively.
Design Philosophy
- Express.js: Express.js is known for its minimalistic design. It provides a lightweight and flexible framework that allows developers to have full control over their application’s structure and architecture.
- Nest.js: Nest.js takes a structured approach. It follows the architectural patterns of Angular and encourages the use of decorators and TypeScript to define modules, controllers, and services.
TypeScript Support
- Express.js: Express.js has no native TypeScript support. However, you can still use TypeScript with Express.js by manually configuring and transpiling the TypeScript code to JavaScript.
- Nest.js: Nest.js is built with TypeScript in mind and has native support for TypeScript. It provides decorators and TypeScript features out of the box, making it easier to build Node.js applications with a strong typing system.
Ultimately, the choice between Express.js and Nest.js depends on your project requirements, team preferences, and the level of structure you desire. If you prefer a minimalistic and flexible framework, Express.js might be the right choice. On the other hand, if you value architectural patterns, strong typing Nest.js can be a great fit.
Quote of the Day
“The best thing about a boolean is even if you are wrong, you are only off by a bit.” — Privately held by me
Thanks for reading! happy coding